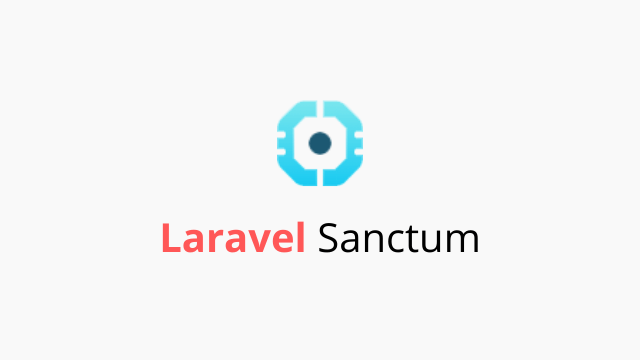
In modern web development, building robust APIs is essential for creating dynamic and interactive applications. However, ensuring the security of these APIs is equally crucial to protect sensitive data and prevent unauthorized access. Laravel, a popular PHP framework, provides developers with powerful tools to create secure APIs effortlessly. One such tool is Laravel Sanctum, which simplifies API authentication while maintaining a high level of security. In this guide, we'll explore how to implement API authentication with Laravel Sanctum.
What is Laravel Sanctum?
Laravel Sanctum is a simple package for API authentication. It offers a lightweight authentication system based on Laravel's built-in authentication features like sessions and tokens. Unlike Laravel Passport, which is a full OAuth2 server implementation, Sanctum is more lightweight and tailored specifically for single-page applications (SPAs), mobile applications, and simple APIs.
Setting Up Laravel Sanctum
To begin using Sanctum in your Laravel project, follow these steps:
1. Install Sanctum: Start by installing Sanctum via Composer:
composer require laravel/sanctum
2. Publish Configuration: Publish the Sanctum configuration file to customize Sanctum's behavior if needed:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
3. Run Migrations: Run the migrations to create the necessary database tables for Sanctum:
php artisan migrate
4. Add Middleware: Ensure that Sanctum's middleware is present in your api middleware group within your app/Http/Kernel.php file:
'api' => [ \Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class, 'throttle:api', \Illuminate\Routing\Middleware\SubstituteBindings::class, ],
Using Sanctum for API Authentication
Now that you have Sanctum set up in your Laravel application, you can start authenticating users for your API endpoints.
Personal Access Tokens
Sanctum provides a simple way to authenticate users using personal access tokens. Here's how you can generate and use personal access tokens:
1. Generate Token: You can generate a personal access token for a user using Sanctum's createToken method:
$token = $user->createToken('token-name')->plainTextToken;
2. Use Token: To authenticate API requests, include the token in the request headers:
Authorization: Bearer {token}
SPA Authentication
For single-page applications (SPAs), Sanctum offers a stateful authentication mechanism using Laravel sessions. This approach allows you to authenticate users using sessions while still making API requests from your SPA.
1. Register Routes: Register the necessary routes for Sanctum's SPA authentication in your routes/api.php file:
Route::middleware('auth:sanctum')->get('/user', function (Request $request) { return $request->user(); });
2. Authenticate User: To authenticate a user, send a POST request to Laravel's /login route with the user's credentials:
$response = $client->post('/login', [ 'email' => 'user@example.com', 'password' => 'password', ]);
3. Access Authenticated Routes: Once authenticated, you can access protected routes by including the session cookie in subsequent requests.
Conclusion
Laravel Sanctum provides a simple yet powerful solution for authenticating users in Laravel APIs. Whether you're building SPAs, mobile applications, or traditional web applications, Sanctum offers the flexibility and security you need to protect your API endpoints. By following the steps outlined in this guide, you can easily integrate Sanctum into your Laravel projects and ensure the security of your API. Happy coding!
Search by keyword
Recent Posts
-
MyRestaurant ALX research project
Jun 12, 2024
-
Demystifying the Journey: What Happens When Y...
Apr 13, 2024
-
Secure Your Laravel API with Sanctum: A Compr...
Mar 23, 2024